Android game framework
In this tutorial I will present a basic game flow and a game framework that I've made and that we will use for making Android games.
It’s good to have a well organized framework so that the code is readable and that you can easier found what you are looking for.
A basic game flow
The diagram below shows a basic game flow that almost all games have.

The game flow in my framework goes like this:
There is no “Launch Game” state in my framework, it goes directly to “Main Menu”. First thing that happens is creation of main activity, which is class MainMenu.java. In AndroidManifest.xml is set, that this class/activity is started first.
The main menu look is designed in res/layout/activity_main_menu.xml file. For now there is only one button, which starts the game when you click it. So, when the button is clicked we enter the “New game” state. In this state we prepare surface for drawing and for touch events, then game loop is started which will execute “Update” and “Draw”.
Framework
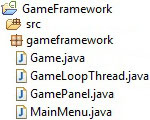
This framework consists of four classes: MainMenu.java, GamePanel.java, GameLoopThread.java and Game.java
First is the “MainMenu.java”, this is activity that is first shown on a screen. We had to set that “MainMenu.java” is launched first in AndroidManifest.xml. In manifest we also set that screen orientation is landscape.
Here is how this activity is set in AndroidManifest.xml:
<activity android:name=“.MainMenu” android:label=“@string/title_activity_main_menu” android:screenOrientation=“landscape” android:theme=“@android:style/Theme.NoTitleBar.Fullscreen” > <intent-filter> <action android:name=“android.intent.action.MAIN” /> <category android:name=“android.intent.category.LAUNCHER” /> </intent-filter> </activity>
Second one is the “GamePanel .java”, here is created a surface for drawing and touch events. The third is the "GameLoopThread .java", this class controls the game, update and draws it on the screen. The last, fourth class is the "Game.java"; this is the actual game.
In the source code (that you can download at the bottom of this page) I commented the code, but here I will explain each class and how the framework works.
MainMenu.java
This class is used for main menu. The main menu look is designed in res/layout/activity_main_menu.xml file. We set this layout as content view in onCreate() method when MainMenu.java activity is started. For now there is only one button in main menu, which starts the game when you click it. In activity_main_menu.xml is set onClick event on this button to call method onClickStartGame(View v) that is in MainMenu.java. When this method is called we set new content view, that is GamePanel.java which extends SurfaceView.
GamePanel.java
This class extends SurfaceView which we will need further for canvas to draw on it. Also this class implements SurfaceHolder.Callback that we will use for touch events. When surface is created we start the game loop in startGame() method. In this class we also create Game.java object, that is given to GameLoopTheread.java object in startGame() method. The reason why we create Game.java object here is because of touch events.
In Game.java we have methods that must be called when a touch event occurs and this is happening in onTouchEvent(MotionEvent event) in GamePanel.java class.
public boolean onTouchEvent(MotionEvent event) { int action = event.getAction(); if(action == MotionEvent.ACTION_DOWN){ game.touchEvent_actionDown(event); } if(action == MotionEvent.ACTION_MOVE) { game.touchEvent_actionMove(event); } if(action == MotionEvent.ACTION_UP){ game.touchEvent_actionUp(event); } return true; }
Here in this method we check what kind of touch action was. There are three kinds of motion events that we use here: ACTION_DOWN, ACTION_MOVE and ACTION_UP. This method is prepared for single-touch, for multi-touch is a bit different. For multi-touch you have to use pointer ids and some other motion events. Action ACTION_DOWN happens when we put our finger on a screen. Action ACTION_MOVE happens when we move this finger that is on a screen. And action ACTION_UP happens last, when we remove finger from the screen.
GameLoopThread.java
This class contains game loop which controls game updates and drawing to the screen. In this loop we call Game.java update and draw methods.
... this.game.Update(this.gameTime); this.game.Draw(canvas); ...
How many times per second are this methods called is set with MAX_FPS constant. This game loop is such that can skip some drawings to a screen and only do the update if short of time, that way it can catch up, so that game speed is constant. You can read more about this game loop in this article titled the Android game loop.
GameLoopThread.java
This class contains an actual game.
In the constructor we save screen width, height and density. Then we call LoadContent(resources) method in which we will load necessary files and we call ResetGame() method which is intent for (re)setting some game variables before game can start.
Into Update(long gameTime) method we will write code for updating the game and into Draw(Canvas canvas) method we will write code that draws the game to the screen.
In this class we have three more methods that are intent for touch events.
/** * When touch on screen is detected. * * @param event MotionEvent */ public void touchEvent_actionDown(MotionEvent event){ } /** * When moving on screen is detected. * * @param event MotionEvent */ public void touchEvent_actionMove(MotionEvent event){ } /** * When touch on screen is released. * * @param event MotionEvent */ public void touchEvent_actionUp(MotionEvent event){ }
Best way to understand all of this is to look at next tutorials and see actual game.
This framework also includes Animation.java class for animating and Sound.java class for sound. I will introduce these two classes in further tutorials.
Download
Here is a zip file which contains project files:
Download Android game framework source code.